python五子棋实验报告
Python五子棋实验报告
Python五子棋是一种基于Python编程语言的人机对战棋类游戏,其实现过程主要包括棋盘绘制、玩家交互、胜负判断等功能。本次实验旨在通过实现Python五子棋游戏,提高学生对Python语言的掌握能力,同时加深对算法思想的理解。
实验过程
1.棋盘绘制
棋盘绘制是实现Python五子棋游戏的第一步,我们通过使用Python的turtle库,绘制出一个19*19的棋盘。具体实现过程如下:
`python
import turtle
# 绘制棋盘
def draw_board():
turtle.speed(0)
turtle.color("black")
turtle.pensize(2)
turtle.penup()
turtle.goto(-180, 180)
turtle.pendown()
for i in range(19):
turtle.forward(360)
turtle.penup()
turtle.goto(-180, turtle.ycor() - 20)
turtle.pendown()
turtle.right(90)
for i in range(19):
turtle.forward(360)
turtle.penup()
turtle.goto(turtle.xcor() - 20, 180)
turtle.pendown()
turtle.hideturtle()
draw_board()
2.玩家交互
玩家交互是Python五子棋游戏的核心,我们需要实现玩家与计算机的交互,即玩家下棋后,计算机判断胜负并进行相应的反应。具体实现过程如下:
`python
# 判断胜负
def is_win(board, x, y, color):
count = 1
# 判断横向
i = 1
while x + i < 19 and board[x + i][y] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and board[x - i][y] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断纵向
count = 1
i = 1
while y + i < 19 and board[x][y + i] == color:
count += 1
i += 1
i = 1
while y - i >= 0 and board[x][y - i] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断左上到右下
count = 1
i = 1
while x + i < 19 and y + i < 19 and board[x + i][y + i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y - i >= 0 and board[x - i][y - i] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断右上到左下
count = 1
i = 1
while x + i < 19 and y - i >= 0 and board[x + i][y - i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y + i < 19 and board[x - i][y + i] == color:
count += 1
i += 1
if count >= 5:
return True
return False
# 玩家下棋
def player_move(board, player_color):
x, y = turtle.numinput("下棋", "请输入坐标(1-19):", default=None, minval=1, maxval=19), turtle.numinput("下棋", "请输入坐标(1-19):", default=None, minval=1, maxval=19)
x, y = int(x) - 1, int(y) - 1
if board[x][y] != 0:
turtle.textinput("提示", "该位置已有棋子,请重新输入。")
player_move(board, player_color)
else:
draw_chess(x, y, player_color)
board[x][y] = player_color
if is_win(board, x, y, player_color):
turtle.textinput("游戏结束", "你赢了!")
turtle.bye()
else:
computer_move(board, 3 - player_color)
# 计算机下棋
def computer_move(board, computer_color):
x, y = get_computer_move(board, computer_color)
draw_chess(x, y, computer_color)
board[x][y] = computer_color
if is_win(board, x, y, computer_color):
turtle.textinput("游戏结束", "你输了!")
turtle.bye()
# 获取计算机下棋位置
def get_computer_move(board, computer_color):
for i in range(19):
for j in range(19):
if board[i][j] == 0:
board[i][j] = computer_color
if is_win(board, i, j, computer_color):
board[i][j] = 0
return i, j
board[i][j] = 0
return get_random_move(board, computer_color)
# 获取随机下棋位置
def get_random_move(board, color):
import random
x, y = random.randint(0, 18), random.randint(0, 18)
while board[x][y] != 0:
x, y = random.randint(0, 18), random.randint(0, 18)
return x, y
# 绘制棋子
def draw_chess(x, y, color):
turtle.speed(0)
turtle.penup()
turtle.goto(x * 20 - 180, 180 - y * 20)
turtle.pendown()
if color == 1:
turtle.color("black")
else:
turtle.color("white")
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
3.胜负判断
胜负判断是Python五子棋游戏的最后一步,我们需要在玩家下棋后,判断当前局面是否有胜负。具体实现过程如下:
`python
# 判断胜负
def is_win(board, x, y, color):
count = 1
# 判断横向
i = 1
while x + i < 19 and board[x + i][y] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and board[x - i][y] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断纵向
count = 1
i = 1
while y + i < 19 and board[x][y + i] == color:
count += 1
i += 1
i = 1
while y - i >= 0 and board[x][y - i] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断左上到右下
count = 1
i = 1
while x + i < 19 and y + i < 19 and board[x + i][y + i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y - i >= 0 and board[x - i][y - i] == color:
count += 1
i += 1
if count >= 5:
return True
# 判断右上到左下
count = 1
i = 1
while x + i < 19 and y - i >= 0 and board[x + i][y - i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y + i < 19 and board[x - i][y + i] == color:
count += 1
i += 1
if count >= 5:
return True
return False
问答
1.如何运行Python五子棋游戏?
运行Python五子棋游戏需要先安装Python编程环境,然后在命令行或集成开发环境中打开游戏文件,运行即可。
2.如何实现玩家与计算机的交互?
玩家与计算机的交互可以通过turtle库实现,具体实现过程包括玩家下棋、计算机下棋、获取计算机下棋位置、获取随机下棋位置等步骤。
3.如何判断胜负?
判断胜负可以通过判断当前局面是否有五个连续的同色棋子实现,具体实现过程包括判断横向、纵向、左上到右下、右上到左下四个方向是否有五个连续的同色棋子。

相关推荐HOT
更多>>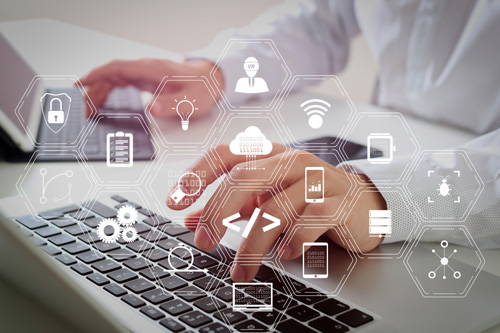
python求最大值的代码
**Python求最大值的代码**在Python中,求最大值是一个常见的任务。Python提供了多种方法来找到一组数据中的最大值,其中包括使用内置的max()函...详情>>
2023-11-16 23:49:21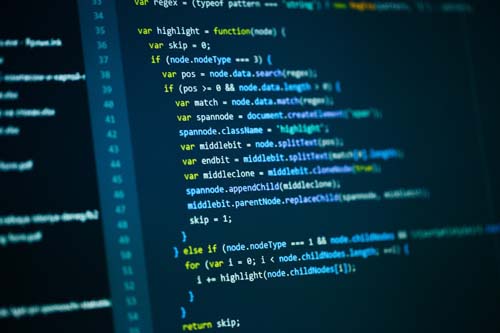
python求1到n的偶数和
Python求1到n的偶数和Python是一种高级编程语言,它被广泛应用于数据分析、人工智能、Web开发等领域。Python语言简单易学,语法简洁明了,因此...详情>>
2023-11-16 22:45:37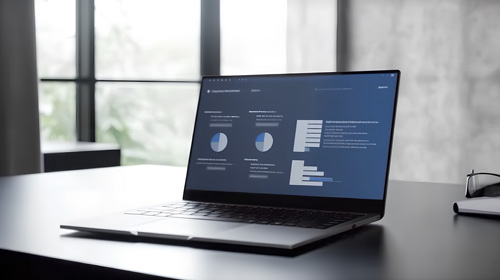
python实训心得体会4000字
Python实训心得体会4000字在Python实训中,我深刻体会到了Python语言的魅力和应用价值。Python是一门简洁、易学、高效的编程语言,具有广泛的应...详情>>
2023-11-16 20:31:51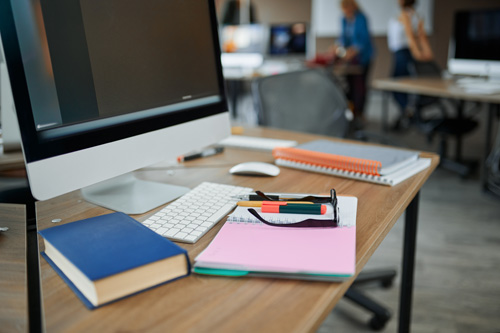
python五子棋实验报告
Python五子棋实验报告Python五子棋是一种基于Python编程语言的人机对战棋类游戏,其实现过程主要包括棋盘绘制、玩家交互、胜负判断等功能。本次...详情>>
2023-11-16 16:51:18