JavaScript全解析——node实现mongodb数据库的操作
问node要实现MongoDB数据库的操作总共分几步?答:6步。
1.和数据库建立连接
/*
数据库的操作步骤
+ 操作 mongodb 数据需要使用第三方
=> mongoose
*/
// 0. 下载第三方并导入
const mongoose = require("mongoose");
/**
* 1. 和数据库建立连接
* 语法: mongoose.connect('数据库的地址', 回调函数)
* 数据库的地址: mongodb://localhost:27017/库名
* 注意: 如果当前数据库内没有该 库, 会创建一个 库 连接进去, 如果有这个 库, 直接连接进去
*/
mongoose.connect("mongodb://localhost:27017/test2203", () =>
console.log("连接数据库成功")
);
2.创建一个表模型, 后续需要利用这个表模型创建出来一个 "遥控器", 用于数据库的增删改查
/**
* 2. 创建一个 表模型(按照表头来建立模型)
* 语法: new mongoose.Schema({ 模型配置 })
* 返回值: 一个表模型
*/
const users = new mongoose.Schema({
nickname: String, // 该表内有一个 nickname 表头, 是 String 类型
age: Number, // 该表内有一个 age 表头, 是 Number 类型
createTime: {
// 该表内 有一个 createTime 表头, 有多条限制
type: Date, // 类型为 Date
default: new Date(), // 默认是当前时间
},
password: {
type: String,
require: true, // 必填
},
hobby: Array,
gender: {
type: String,
enum: ["男", "女", "不确定"], // 枚举, 该属性的值只能是该数组内的成员, 其他的不行
},
});
3.利用表模型创建一个 "遥控器"
a.注意:
i.如果该库内有该表, 那么直接使用, 如果没有该表, 那么会按照 表模型创建一个表 在使用
ii.表名尽量使用 复数形式
const UsersModel = mongoose.model("users", users);
4.利用遥控器去 存储一条数据
new UsersModel({
nickname: "管理员",
age: 18,
password: "123456",
hobby: ["足球", "篮球"],
gender: "男",
})
.save()
.then((res) => {
console.log("存储数据成功", res);
})
.catch((err) => {
console.log("存储数据失败", err);
});
5.利用遥控器去查询数据库
/**
* 5.1 基础查询
* 语法: UsersModel.find()
* 结果: 查询该表内所有的数据, 以数组的形式返回所有数据
*/
// UsersModel.find().then((res) => {
// console.log(res);
// });
/**
* 5.2 条件查询
* 语法: UsersModel.find({ 条件 })
*/
// UsersModel.find({
// age: 20, // age === 20
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// age: { $gt: 20 }, // age > 20
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// age: { $lt: 20 }, // age < 20
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// age: { $gt: 20, $lt: 23 }, // age > 20 && age < 23
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// nickname: /三/
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// nickname: /三$/,
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// nickname: /三$/,
// age: 20
// }).then((res) => {
// console.log(res, res.length);
// });
// UsersModel.find({
// username: "前端传递过来的username",
// password: "前端传递过来的password",
// }).then((res) => {
// console.log(res, res.length);
// });
/**
* 5-3 分页查询
* 语法: UsersModel.find().skip(开始索引).limit(多少个)
*/
// UsersModel.find()
// .skip(0)
// .limit(20)
// .then((res) => {
// console.log(res, res.length);
// });
/**
* 5-4 根据 ID 查找
* UsersModel.findById(id)
* 注意: 如果查询到了该 id 匹配的数据, 那么就是该数据(对象形式)
* 如果没有查询到该 id, 那么返回一个 null
*/
UsersModel.findById("638e0d959b9b0b7359a89e38").then((res) => {
console.log(res);
});
6.删改数据
// 6. 修改数据
/**
* 6-1 修改一个数据
* 找到满足条件的第一个数据修改
*/
// UsersModel.updateOne({ gender: "不确定" }, { nickname: "QF001" }).then(
// (res) => {
// console.log(res);
// }
// );
/**
* 6-2 修改多个值
* 有多少满足条件的就修改多少个
*/
// UsersModel.updateMany({ gender: "不确定" }, { nickname: "QF001" }).then(
// (res) => {
// console.log(res);
// }
// );
// UsersModel.find({
// gender: "不确定",
// }).then(res => {
// console.log(res)
// });
/**
* 6-3 根据 ID 修改数据
* 找到对应的 ID, 然后修改
*
* 注意, 他的流程是, 先找到对应的值, 然后返回出来的同时, 会将数据修改掉, 但返回来的数据是修改之前的数据
*/
// UsersModel.findByIdAndUpdate("638e0d959b9b0b7359a89e25", {
// nickname: "QF999",
// }).then((res) => console.log(res));
// 7. 删除操作
// 7-1 删除一个数据
// UsersModel.deleteOne({ gender: "不确定" }).then((res) => console.log(res));
// 7-2 删除多个数据
// UsersModel.deleteMany({ gender: "不确定" }).then((res) => console.log(res));
// 7-3 根据 ID 删除数据
// UsersModel.findByIdAndDelete("638e0d959b9b0b7359a89e29").then((res) =>
// console.log(res)
// );

猜你喜欢LIKE
相关推荐HOT
更多>>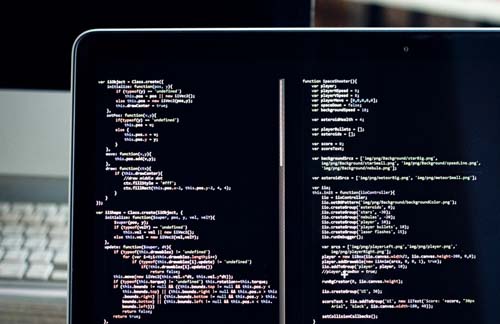
servlet底层原理是什么?
1、ServletAPI核心类与接口2、Servlet类处理请求的流程创建servlet类的步骤:创建一个命名为TestServlet继承javax.servlet.http.HttpServlet类详情>>
2023-05-30 10:41:22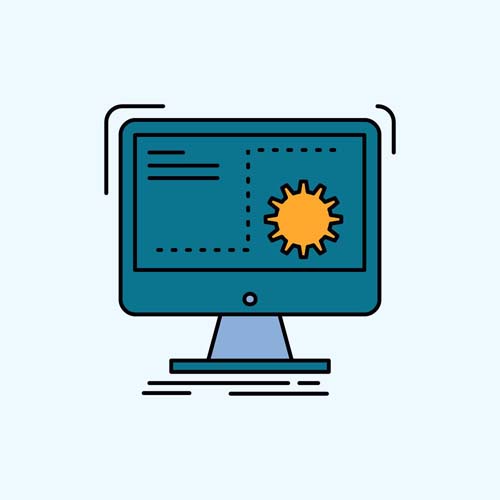
多线程的优势与劣势分别是什么?
多线程是指在同一个程序中,同时运行多个线程,每个线程都可以独立执行不同的任务,相互之间不会干扰。多线程的优势和劣势如下:优势:提高程序...详情>>
2023-05-30 10:32:12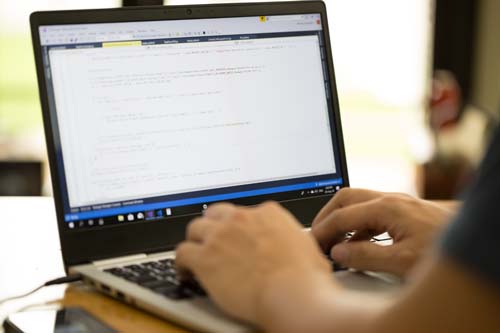
设计模式之生产者与消费者的代码实现
本文主要讲述生产者和消费者模式,文中会使用通俗易懂的案例,使你更好地学习本章知识点并理解原理,做到有道无术。什么是生产者和消费者模式生...详情>>
2023-05-30 10:25:46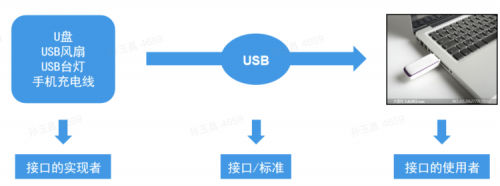
从零开始学Java之interface接口
一.接口简介简介Java中的接口(interface)类似于是一种特殊的抽象类,它也是众多抽象方法的集合。接口的定义方式、组成部分都与抽象类相似,却比...详情>>
2023-05-29 11:26:17热门推荐
如何进行mysql数据备份?
沸什么是servlet的生命周期?servlet请求处理流程是怎样的?
热servlet底层原理是什么?
热怎样编写java程序?
新多线程的优势与劣势分别是什么?
ssm框架的作用与原理是什么?
设计模式之生产者与消费者的代码实现
接口和抽象类有什么区别?4个方面对比
从零开始学Java之interface接口
从零开始学Java之Java中的内部类是怎么回事?
一分钟带你了解MySQL——基础与介绍
在java中,super关键字怎样使用
什么是事件流以及事件流的传播机制 ?
弹性盒有哪些属性是在父元素身上?
技术干货
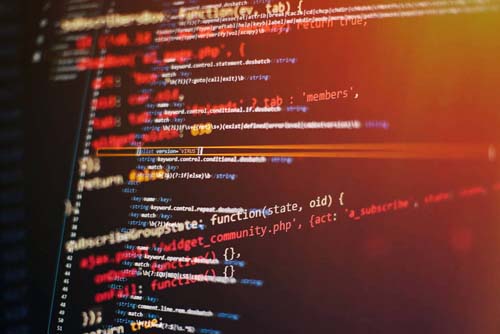
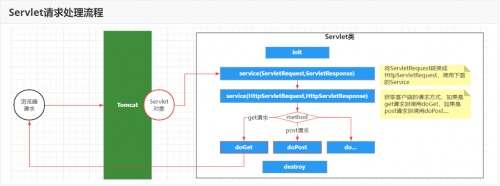
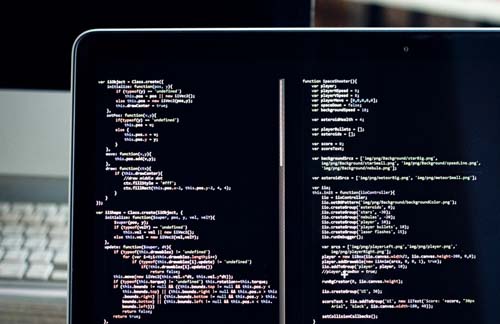
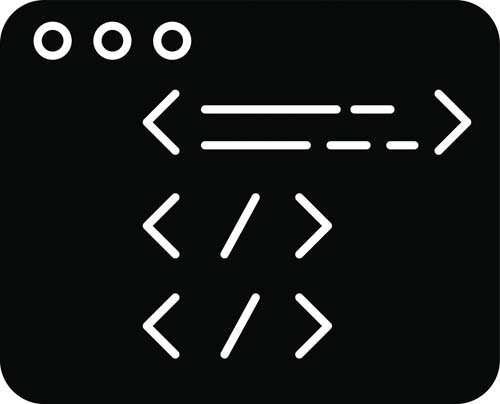
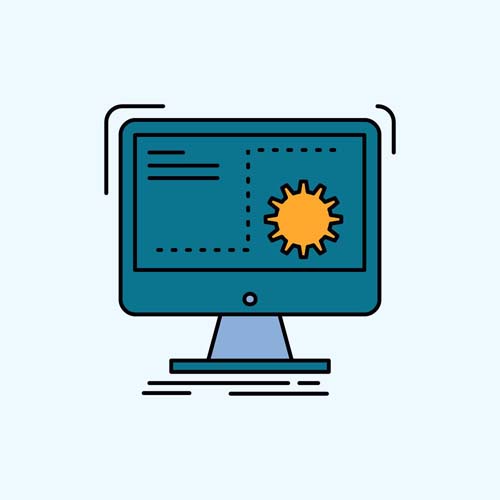
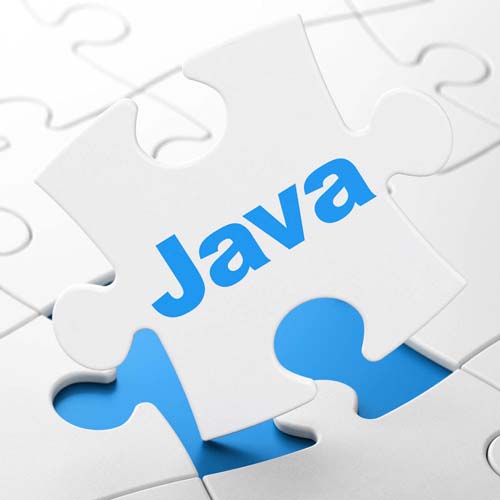
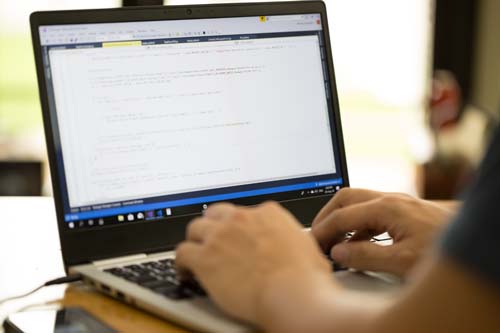