JavaScript全解析之面向对象——判断数据类型
typeof
●用来判断数据类型的
●但是只能准确的判断基本数据类型
constructor
●语法: 数据结构.constructor
●返回值: 该数据结构所属的构造函数
●缺点:
○undefined 和 null 出不来
console.log(p.constructor)
console.log([].constructor)
console.log((function () {}).constructor)
console.log(/^$/.constructor)
// 判断一个数据是不是数组
console.log([].constructor === Array)
instanceOf
●语法: 数据结构 instanceof 构造函数
●得到: 一个布尔值
●缺点:
○undefined 和 null 出不来
function Person() {}
const p = new Person()
console.log(p instanceof Person)
console.log(p instanceof Array)
console.log([] instanceof Array)
Object.prototype.toString.call
●语法: Object.prototype.toString.call(你要判断的数据结构)
●返回值: '[object 数据类型]'
●可以准确的判断所有数据类型
console.log(Object.prototype.toString.call({}))
console.log(Object.prototype.toString.call([]))
console.log(Object.prototype.toString.call(123))
console.log(Object.prototype.toString.call('123'))
console.log(Object.prototype.toString.call(true))
console.log(Object.prototype.toString.call(undefined))
console.log(Object.prototype.toString.call(null))
console.log(Object.prototype.toString.call(new Date()))
console.log(Object.prototype.toString.call(/^$/))
console.log(Object.prototype.toString.call(function () {}))
console.log(Object.prototype.toString.call(function () {}) === '[object Object]')
案例--轮播图
结构
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="./index.css">
</head>
<body>
<!-- HTML 结构 -->
<div class="banner" id="banner">
<ul class="imgBox">
<li class="active" style="background-color: skyblue;">1</li>
<li style="background-color: orange;">2</li>
<li style="background-color: purple;">3</li>
<li style="background-color: green;">4</li>
<li style="background-color: cyan;">5</li>
</ul>
<ol class="pointBox"></ol>
<div class="prev"><</div>
<div class="next">></div>
</div>
<script src="./swiper.js"></script>
<script>
// 实现轮播图
new Banner('#banner', { duration: 2000 })
</script>
</body>
</html>
样式
* {
margin: 0;
padding: 0;
}
ul, ol, li {
list-style: none;
}
.banner {
width: 600px;
height: 400px;
border: 4px solid pink;
margin: 50px auto;
position: relative;
}
.banner > .imgBox {
width: 100%;
height: 100%;
position: relative;
}
.banner > .imgBox > li {
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
display: flex;
justify-content: center;
align-items: center;
font-size: 100px;
color: #fff;
opacity: 0;
transition: all .3s linear;
}
.banner > .imgBox > li.active {
opacity: 1;
}
.banner > .pointBox {
width: 200px;
height: 30px;
background-color: rgba(0, 0, 0, .5);
border-radius: 15px;
position: absolute;
left: 50%;
bottom: 30px;
transform: translateX(-50%);
display: flex;
justify-content: space-evenly;
align-items: center;
}
.banner > .pointBox > li {
width: 20px;
height: 20px;
border-radius: 50%;
background-color: #fff;
cursor: pointer;
}
.banner > .pointBox > li.active {
background-color: red;
}
.banner > div {
width: 30px;
height: 50px;
position: absolute;
top: 50%;
transform: translateY(-50%);
background-color: rgba(0, 0, 0, .5);
display: flex;
justify-content: center;
align-items: center;
font-size: 20px;
font-weight: 700;
cursor: pointer;
color: #fff;
}
.banner > div.prev {
left: 0;
}
.banner > div.next {
right: 0;
}
交互
// 面向对象的方式书写 轮播图的代码
/*
分析:
+ 属性
=> banner: 可视区域
=> imgBox: 承载图片的大盒子
=> pointBox: 承载焦点的大盒子
=> index: 表示当前第几张
=> timer: 定时器返回值
+ 方法
=> 设置焦点
分析:
+ 自动轮播: 每间隔 2000ms 切换下一张
+ 功能:
=> 上一张: 当前这一张取消 active, 上一张 添加 active
=> 下一张: 当前这一张取消 active, 下一张 添加 active
=> 某一张: 当前这一张取消 active, 某一张 添加 active
+ 功能:
=> 某一张: 当前这一张取消 active, 某一张 添加 active
=> 有的时候, 某一张是 index++
=> 有的时候, 某一张是 index--
=> 有的时候, 某一张是 index = xxx
*/
// 轮播图 类
class Banner {
constructor (selector, options = {}) {
// 获取可视区域
this.banner = document.querySelector(selector)
// 承载图片的盒子
this.imgBox = this.banner.querySelector('.imgBox')
// 承载焦点的盒子
this.pointBox = this.banner.querySelector('.pointBox')
// 准备变量表示当前第几张
this.index = 0
// 准备变量接受定时器返回值
this.timer = 0
this.options = options
// 调用方法
this.setPoint()
this.autoPlay()
this.overOut()
this.bindEvent()
}
// 书写方法
setPoint () {
// 1. 拿到有多少个焦点需要生成
const pointNum = this.imgBox.children.length
// 2. 循环生成
for (let i = 0; i < pointNum; i++) {
const li = document.createElement('li')
li.classList.add('item')
// 第一个 li 有 active 类名
if (i === 0) li.classList.add('active')
li.dataset.point = i
this.pointBox.appendChild(li)
}
}
// 切换一张的方法
// 给 changeOne 设置一个参数
// true, false, 数字
// true, 表示下一张
// false, 上一张
// 数字, 某一张
changeOne (type) {
// index 表示的就是当前的索引
this.imgBox.children[this.index].classList.remove('active')
this.pointBox.children[this.index].classList.remove('active')
// 调整 index
if (type === true) {
this.index++
} else if (type === false) {
this.index--
} else {
this.index = type
}
// 判断一下 index 的边界
if (this.index >= this.imgBox.children.length) this.index = 0
if (this.index < 0) this.index = this.imgBox.children.length - 1
// 让当前这一个显示
this.imgBox.children[this.index].classList.add('active')
this.pointBox.children[this.index].classList.add('active')
}
// 自动轮播
autoPlay () {
this.timer = setInterval(() => {
// 下一张
this.changeOne(true)
}, this.options.duration || 5000)
}
// 移入移出
overOut () {
this.banner.addEventListener('mouseover', () => clearInterval(this.timer))
this.banner.addEventListener('mouseout', () => this.autoPlay())
}
// 点击事件
bindEvent() {
this.banner.addEventListener('click', e => {
if (e.target.className === 'prev') this.changeOne(false)
if (e.target.className === 'next') this.changeOne(true)
if (e.target.className === 'item') this.changeOne(e.target.dataset.point - 0)
})
}
}

猜你喜欢LIKE
相关推荐HOT
更多>>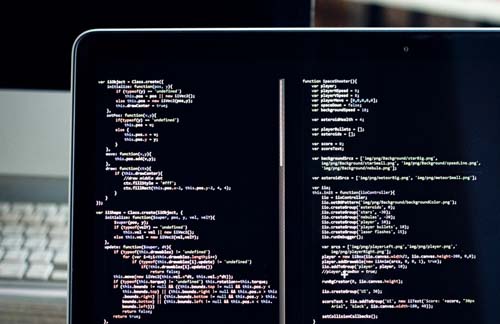
servlet底层原理是什么?
1、ServletAPI核心类与接口2、Servlet类处理请求的流程创建servlet类的步骤:创建一个命名为TestServlet继承javax.servlet.http.HttpServlet类详情>>
2023-05-30 10:41:22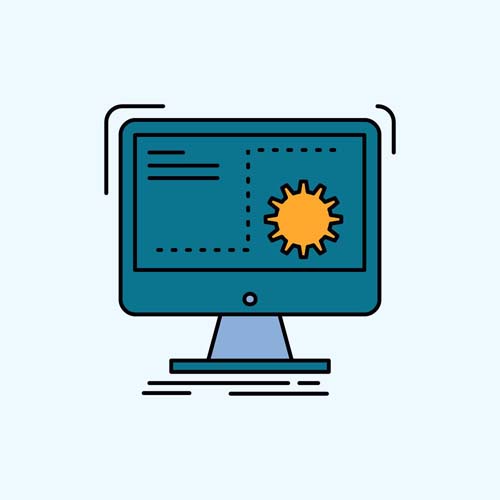
多线程的优势与劣势分别是什么?
多线程是指在同一个程序中,同时运行多个线程,每个线程都可以独立执行不同的任务,相互之间不会干扰。多线程的优势和劣势如下:优势:提高程序...详情>>
2023-05-30 10:32:12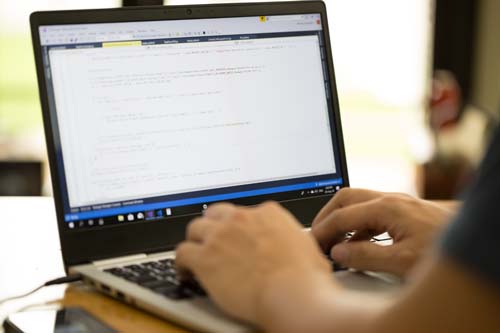
设计模式之生产者与消费者的代码实现
本文主要讲述生产者和消费者模式,文中会使用通俗易懂的案例,使你更好地学习本章知识点并理解原理,做到有道无术。什么是生产者和消费者模式生...详情>>
2023-05-30 10:25:46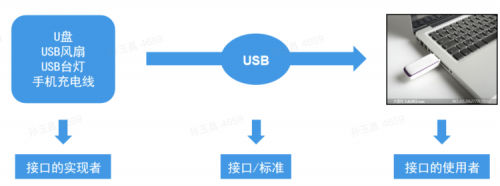
从零开始学Java之interface接口
一.接口简介简介Java中的接口(interface)类似于是一种特殊的抽象类,它也是众多抽象方法的集合。接口的定义方式、组成部分都与抽象类相似,却比...详情>>
2023-05-29 11:26:17热门推荐
如何进行mysql数据备份?
沸什么是servlet的生命周期?servlet请求处理流程是怎样的?
热servlet底层原理是什么?
热怎样编写java程序?
新多线程的优势与劣势分别是什么?
ssm框架的作用与原理是什么?
设计模式之生产者与消费者的代码实现
接口和抽象类有什么区别?4个方面对比
从零开始学Java之interface接口
从零开始学Java之Java中的内部类是怎么回事?
一分钟带你了解MySQL——基础与介绍
在java中,super关键字怎样使用
什么是事件流以及事件流的传播机制 ?
弹性盒有哪些属性是在父元素身上?
技术干货
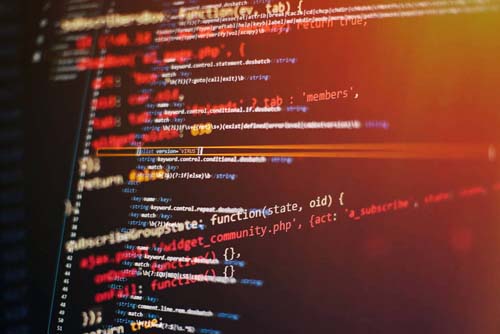
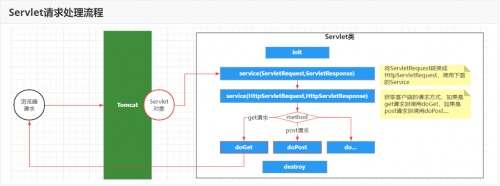
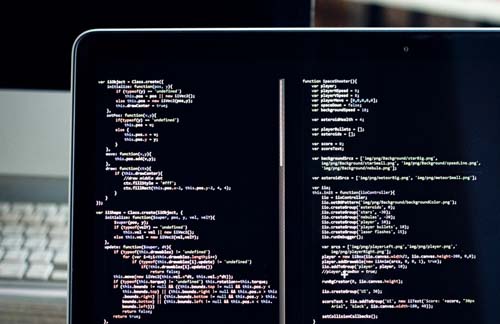
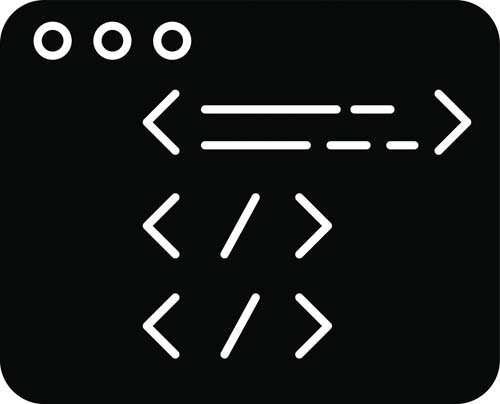
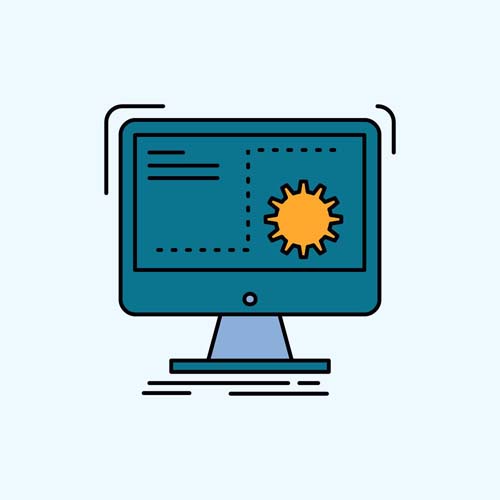
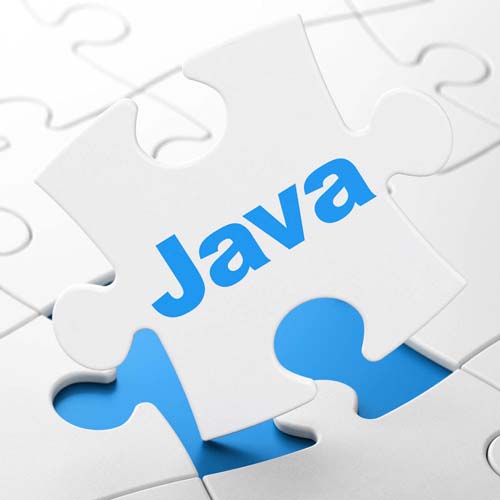
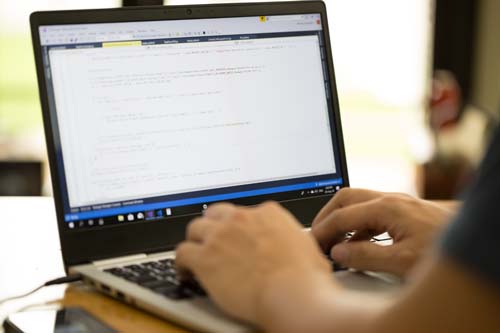